Are you ready to dive deeper into Django development? This complete beginner’s guide to django part 2 builds upon the foundations covered previously, offering hands-on experience with models, views, and templates. At CONDUCT.EDU.VN, we’re dedicated to simplifying complex topics, empowering you to build robust web applications. You’ll master Django fundamentals while creating a functional discussion board, including data persistence, template rendering, and basic testing.
1. Understanding the Web Board Project
Before diving into code, let’s visualize the discussion board we will create. Understanding the project’s structure and user interactions is crucial for a successful development process.
1.1. Use Case Diagram: Defining User Interactions
A use case diagram illustrates how users interact with the system. In our forum, we distinguish between regular users and administrators. Admins can create new boards, while all users can create topics and post replies within those boards.
- Admin User: Creates Boards, Manages Users, Monitors Content.
- Regular User: Creates Topics, Posts Replies, Views Boards and Topics.
1.2. Class Diagram: Structuring the Data
The class diagram represents the entities in our application and their relationships. Key entities include Board, Topic, Post, and User.
- Board: Represents a category for discussions.
- Topic: Represents a single discussion thread within a board.
- Post: Represents a reply within a topic.
- User: Represents a user of the discussion board.
1.3. Detailed Model Attributes
Each model contains specific attributes that define the data they hold:
- Board:
name
(unique),description
. - Topic:
subject
,last_updated
,board
(ForeignKey to Board),starter
(ForeignKey to User). - Post:
message
,created_at
,updated_at
,created_by
(ForeignKey to User),updated_by
(ForeignKey to User, nullable).
1.4. Associations Between Models
The relationships between models are critical for data integrity:
- Board to Topic: A Board can have multiple Topics; a Topic belongs to one Board.
- Topic to Post: A Topic can have multiple Posts; a Post belongs to one Topic.
- Topic to User: A Topic is started by one User; a User can start multiple Topics.
- Post to User: A Post is created by one User and can be updated by one User.
1.5. Wireframes: Visualizing the User Interface
Wireframes are basic visual representations of the user interface. They help define the layout and functionality of each page. Key wireframes include:
- Homepage: Lists all available boards.
- Board Page: Lists all topics within a specific board.
- New Topic Page: Form for creating a new topic.
- Topic Page: Displays posts within a topic and allows users to reply.
- Reply Page: Form for posting a reply to a topic.
2. Defining Models in Django
Models are Python classes that represent database tables. Django’s ORM (Object-Relational Mapper) allows you to interact with the database using Python code instead of raw SQL.
2.1. Creating the Board Model
The Board
model represents a discussion forum category.
from django.db import models
class Board(models.Model):
name = models.CharField(max_length=30, unique=True)
description = models.CharField(max_length=100)
def __str__(self):
return self.name
name
: ACharField
to store the name of the board (maximum length 30 characters, must be unique).description
: ACharField
to store a brief description of the board (maximum length 100 characters).__str__
: Returns thename
of the board as a human-readable string representation.
2.2. Creating the Topic Model
The Topic
model represents a discussion thread within a board.
from django.db import models
from django.contrib.auth.models import User
class Topic(models.Model):
subject = models.CharField(max_length=255)
last_updated = models.DateTimeField(auto_now_add=True)
board = models.ForeignKey(Board, related_name='topics', on_delete=models.CASCADE)
starter = models.ForeignKey(User, related_name='topics', on_delete=models.CASCADE)
def __str__(self):
return self.subject
subject
: ACharField
to store the subject of the topic (maximum length 255 characters).last_updated
: ADateTimeField
that automatically updates when the topic is created.board
: AForeignKey
to theBoard
model, establishing a relationship between a topic and its board.on_delete=models.CASCADE
ensures that if aBoard
is deleted, all associatedTopic
objects are also deleted.starter
: AForeignKey
to theUser
model, indicating the user who started the topic.on_delete=models.CASCADE
ensures that if aUser
is deleted, all associatedTopic
objects are also deleted.__str__
: Returns thesubject
of the topic as a human-readable string representation.
2.3. Creating the Post Model
The Post
model represents a reply within a topic.
from django.db import models
from django.contrib.auth.models import User
class Post(models.Model):
message = models.TextField(max_length=4000)
topic = models.ForeignKey(Topic, related_name='posts', on_delete=models.CASCADE)
created_at = models.DateTimeField(auto_now_add=True)
updated_at = models.DateTimeField(null=True)
created_by = models.ForeignKey(User, related_name='posts', on_delete=models.CASCADE)
updated_by = models.ForeignKey(User, null=True, related_name='+', on_delete=models.SET_NULL)
def __str__(self):
return self.message[:50] # Display the first 50 characters of the message
message
: ATextField
to store the content of the post (maximum length 4000 characters).topic
: AForeignKey
to theTopic
model, establishing a relationship between a post and its topic.on_delete=models.CASCADE
ensures that if aTopic
is deleted, all associatedPost
objects are also deleted.created_at
: ADateTimeField
that automatically sets the current date and time when the post is created.updated_at
: ADateTimeField
that stores the date and time when the post was last updated (can be null if the post has never been updated).created_by
: AForeignKey
to theUser
model, indicating the user who created the post.on_delete=models.CASCADE
ensures that if aUser
is deleted, all associatedPost
objects are also deleted.updated_by
: AForeignKey
to theUser
model, indicating the user who last updated the post.null=True
allows the field to be empty.on_delete=models.SET_NULL
ensures that if aUser
is deleted, theupdated_by
field is set to null instead of deleting the post.__str__
: Returns the first 50 characters of themessage
as a human-readable string representation.
2.4. Understanding Model Fields
Django provides a variety of field types for defining model attributes:
Field Type | Description |
---|---|
CharField |
A character string field with a maximum length. |
TextField |
A large text field. |
IntegerField |
An integer field. |
BooleanField |
A boolean field (True/False). |
DateTimeField |
A date and time field. |
ForeignKey |
A field that represents a one-to-many relationship with another model. |
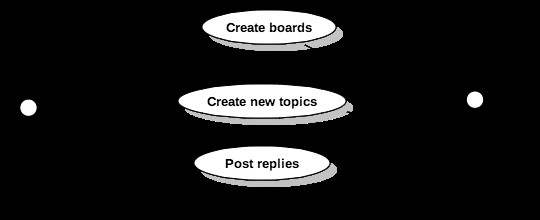
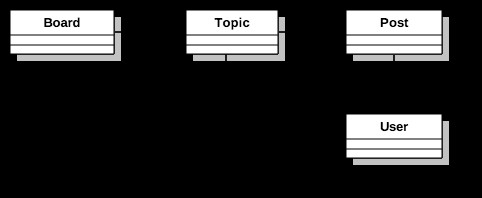
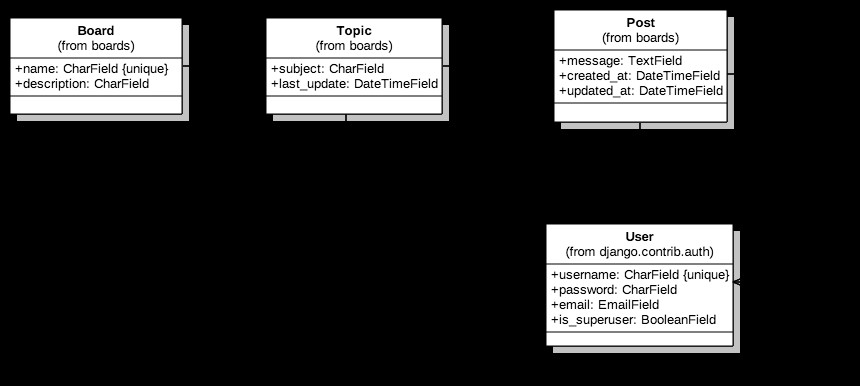
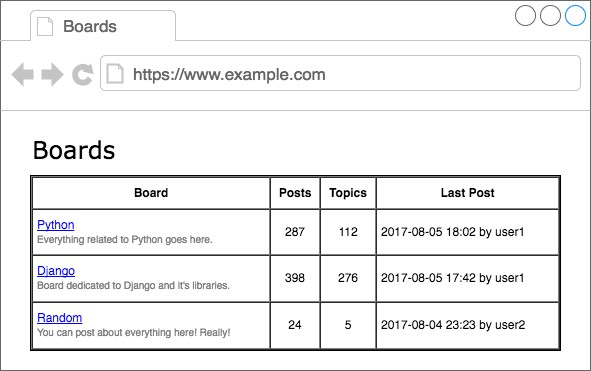
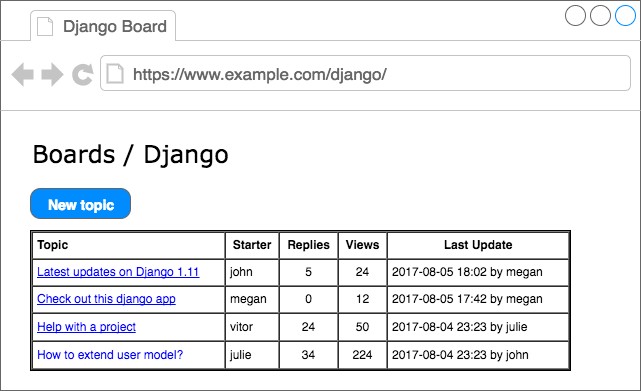
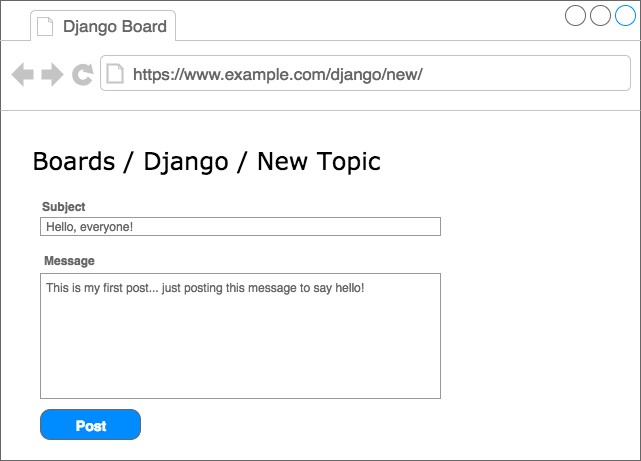
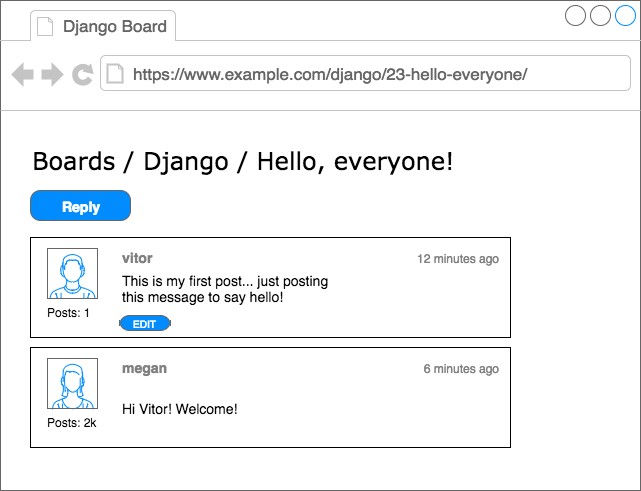
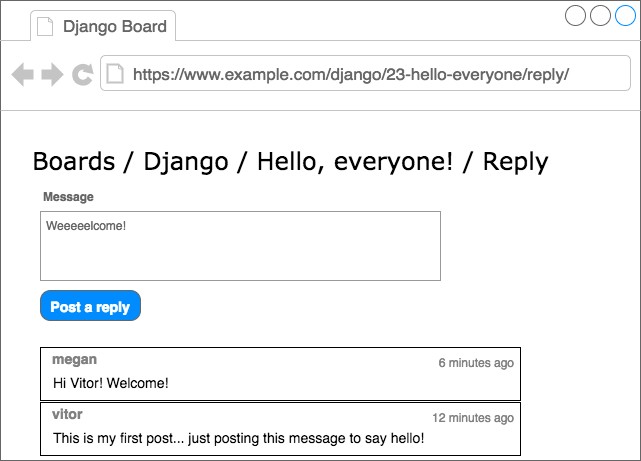
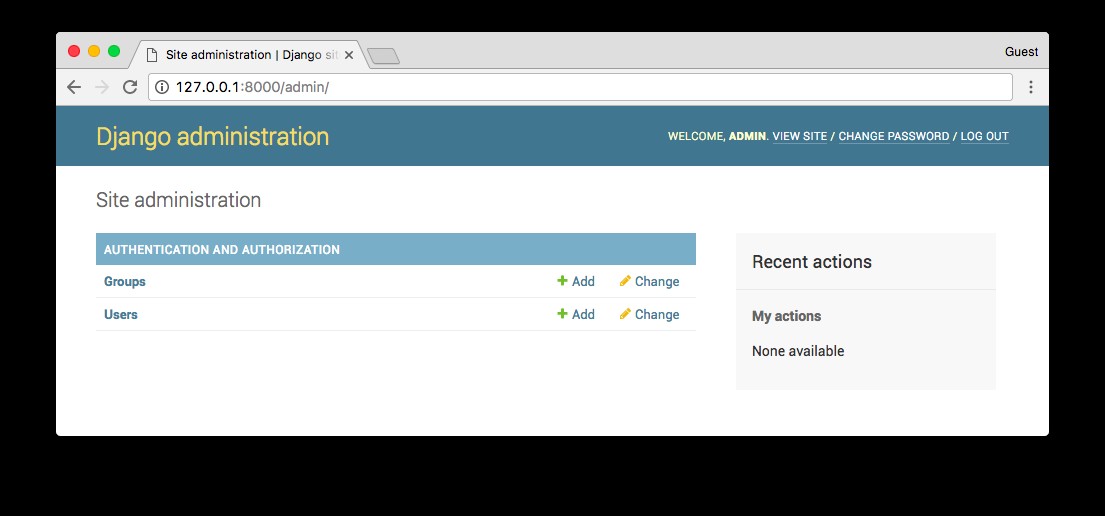
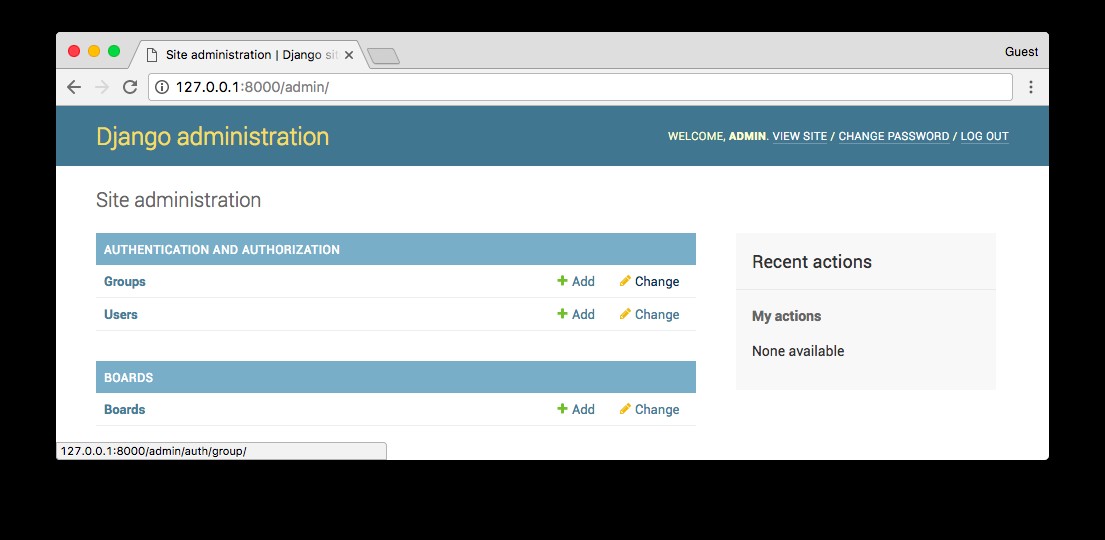
2.5. Key Model Concepts
- ORM (Object-Relational Mapper): Django’s ORM allows you to interact with databases using Python objects.
- Model Fields: Attributes that define the data stored in each model.
- Relationships: Define how models relate to each other (e.g., one-to-many, many-to-many).
3. Migrating the Models
Migrations are how Django propagates changes you make to your models (adding a field, deleting a model, etc.) into your database schema.
3.1. Creating Migrations
Run the following command to create migrations for your models:
python manage.py makemigrations
This command analyzes your models and creates migration files that describe the changes to be applied to the database.
3.2. Applying Migrations
Apply the migrations to update your database schema:
python manage.py migrate
This command executes the migration files, creating the tables and columns defined in your models.
3.3. Examining Migrations
You can view the SQL statements that will be executed by a migration using:
python manage.py sqlmigrate boards 0001
Replace boards
with the name of your app and 0001
with the migration number.
4. Interacting with Models: The Django Shell
The Django shell is an interactive Python interpreter that allows you to interact with your Django project and models directly.
4.1. Starting the Shell
Open the Django shell using:
python manage.py shell
4.2. Creating Objects
Create new objects using the model class:
from boards.models import Board
board = Board(name='Django', description='This is a board about Django.')
board.save() # Save to the database
Or using the create()
method:
board = Board.objects.create(name='Python', description='General discussion about Python.')
4.3. Retrieving Objects
Retrieve all objects of a model:
boards = Board.objects.all()
Retrieve a single object by its primary key (ID):
django_board = Board.objects.get(id=1)
Retrieve a single object by another field:
django_board = Board.objects.get(name='Django')
4.4. Updating Objects
Update an existing object by modifying its attributes and calling save()
:
board = Board.objects.get(name='Django')
board.description = 'Django discussion board.'
board.save()
4.5. Deleting Objects
Delete an object using the delete()
method:
board = Board.objects.get(name='Python')
board.delete()
5. Views, Templates, and Static Files
Views handle the logic of your application, templates define the presentation, and static files provide the styling and assets.
5.1. Creating Views
Views are Python functions that take a web request and return a web response.
from django.shortcuts import render
from .models import Board
def home(request):
boards = Board.objects.all()
return render(request, 'home.html', {'boards': boards})
This view retrieves all boards from the database and passes them to the home.html
template.
5.2. Configuring Templates
Django templates are HTML files with embedded code that allows dynamic content generation.
5.2.1. Creating a Template Directory
Create a directory named templates
in your project root:
myproject/
├── boards/
├── myproject/
├── templates/ # Create this directory
├── manage.py
5.2.2. Adding Template Settings
Update the TEMPLATES
setting in settings.py
:
import os
TEMPLATES = [
{
'BACKEND': 'django.template.backends.django.DjangoTemplates',
'DIRS': [os.path.join(BASE_DIR, 'templates')],
'APP_DIRS': True,
'OPTIONS': {
'context_processors': [
'django.template.context_processors.debug',
'django.template.context_processors.request',
'django.contrib.auth.context_processors.auth',
'django.contrib.messages.context_processors.messages',
],
},
},
]
5.2.3. Creating a Template
Create a template file named home.html
in the templates
directory:
{% load static %}
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Boards</title>
<link rel="stylesheet" href="{% static 'css/bootstrap.min.css' %}">
</head>
<body>
<div class="container">
<ol class="breadcrumb my-4">
<li class="breadcrumb-item active">Boards</li>
</ol>
<table class="table">
<thead class="thead-inverse">
<tr>
<th>Board</th>
<th>Posts</th>
<th>Topics</th>
<th>Last Post</th>
</tr>
</thead>
<tbody>
{% for board in boards %}
<tr>
<td>
{{ board.name }}
<small class="text-muted d-block">{{ board.description }}</small>
</td>
<td class="align-middle">0</td>
<td class="align-middle">0</td>
</tr>
{% endfor %}
</tbody>
</table>
</div>
</body>
</html>
5.3. Configuring Static Files
Static files are assets such as CSS, JavaScript, and images.
5.3.1. Creating a Static Directory
Create a directory named static
in your project root:
myproject/
├── boards/
├── myproject/
├── templates/
├── static/ # Create this directory
│ └── css/ # Create a css directory inside static
├── manage.py
5.3.2. Adding Static Files Settings
Update the STATIC_URL
and STATICFILES_DIRS
settings in settings.py
:
import os
STATIC_URL = '/static/'
STATICFILES_DIRS = [
os.path.join(BASE_DIR, 'static'),
]
5.3.3. Using Static Files
In your templates, use the {% static %}
template tag to link to static files:
{% load static %}
<link rel="stylesheet" href="{% static 'css/bootstrap.min.css' %}">
Download Bootstrap from getbootstrap.com and place the bootstrap.min.css
file in the static/css/
directory.
6. Testing Your Homepage
Testing is essential for ensuring the reliability of your application.
6.1. Writing Tests
Create tests in boards/tests.py
:
from django.core.urlresolvers import reverse
from django.urls import resolve
from django.test import TestCase
from .views import home
class HomeTests(TestCase):
def test_home_view_status_code(self):
url = reverse('home')
response = self.client.get(url)
self.assertEquals(response.status_code, 200)
def test_home_url_resolves_home_view(self):
view = resolve('/')
self.assertEquals(view.func, home)
6.2. Running Tests
Run your tests using:
python manage.py test
These tests verify that the homepage returns a successful status code and that the correct view is used for the root URL.
7. Introduction to Django Admin
The Django admin interface provides a built-in way to manage your models.
7.1. Creating a Superuser
Create an administrative user:
python manage.py createsuperuser
7.2. Registering Models
Register your models in boards/admin.py
:
from django.contrib import admin
from .models import Board, Topic, Post
admin.site.register(Board)
admin.site.register(Topic)
admin.site.register(Post)
Now you can access the admin interface at http://127.0.0.1:8000/admin/
and manage your models.
8. Advanced Django Concepts
8.1. Model Relationships
Understanding model relationships is crucial for designing efficient and scalable Django applications. Let’s explore the different types of relationships:
- One-to-Many: A
ForeignKey
field defines a one-to-many relationship. For example, oneBoard
can have manyTopic
objects. - Many-to-Many: The
ManyToManyField
field defines a many-to-many relationship. For example, aPost
might be associated with multiple tags, and a tag can be associated with multiplePost
objects. - One-to-One: The
OneToOneField
field defines a one-to-one relationship. This is typically used when one model extends another model by adding additional information.
8.2. QuerySets: Efficient Data Retrieval
QuerySets are at the heart of Django’s ORM. They allow you to retrieve data from the database in an efficient and optimized manner. Here are some key concepts:
- Lazy Evaluation: QuerySets are lazy, meaning they are not evaluated until you actually need the data.
- Chaining: You can chain multiple filters and operations on a QuerySet to refine your query.
- Caching: Django caches the results of QuerySets, so subsequent requests for the same data are faster.
8.3. Template Inheritance
Template inheritance is a powerful feature that allows you to create reusable templates and avoid duplication. Here’s how it works:
- Base Template: Define a base template with common elements such as the HTML structure, CSS links, and navigation.
- Child Templates: Create child templates that inherit from the base template and override specific sections.
8.4. Form Handling: User Input
Forms are essential for handling user input. Django provides a robust forms API that simplifies form creation, validation, and processing. Here’s how to use forms:
- Form Class: Define a form class that specifies the fields and validation rules.
- Template Rendering: Render the form in a template, allowing users to input data.
- Data Validation: Validate the form data when it is submitted, ensuring that it meets the specified requirements.
- Data Processing: Process the form data after validation, saving it to the database or performing other actions.
8.5. Middleware: Request and Response Processing
Middleware is a framework of hooks into Django’s request/response processing. It allows you to modify the request and response objects, perform authentication, and implement other cross-cutting concerns.
9. Setting Up URL Routing
URLs are a crucial aspect of web applications. They define the structure of your site and how users access different parts of it. Django’s URL dispatcher provides a powerful and flexible way to map URLs to views.
9.1. Understanding urls.py
Each Django project and app has a urls.py
file that defines the URL patterns. The URL patterns map URLs to specific views.
9.2. Basic URL Patterns
A basic URL pattern consists of a regular expression and a view function. The regular expression matches the URL, and the view function handles the request.
9.3. Named URLs
Named URLs provide a way to refer to URLs in your templates and views without hardcoding the URL patterns. This makes it easier to update URLs without breaking your application.
9.4. Including Other URLconfs
You can include other urls.py
files in your project’s urls.py
file. This allows you to organize your URLs into logical groups.
9.5. Passing Arguments in URLs
You can pass arguments in URLs to your views. This allows you to create dynamic URLs that depend on user input or other data.
10. Optimizing Your Django Application for SEO
Search Engine Optimization (SEO) is the practice of optimizing your website to rank higher in search engine results. Here are some tips for optimizing your Django application for SEO:
- Use Descriptive URLs: Use descriptive and keyword-rich URLs that accurately reflect the content of your pages.
- Optimize Page Titles and Meta Descriptions: Craft compelling page titles and meta descriptions that include relevant keywords.
- Use Header Tags: Use header tags (H1, H2, H3, etc.) to structure your content and highlight important keywords.
- Optimize Images: Optimize images by using descriptive file names and alt attributes.
- Use Internal and External Links: Use internal links to connect related pages on your site, and use external links to link to authoritative sources.
- Create a Sitemap: Create a sitemap and submit it to search engines.
- Use Schema Markup: Use schema markup to provide search engines with more information about your content.
- Ensure Mobile-Friendliness: Ensure that your website is mobile-friendly and responsive.
- Optimize Page Speed: Optimize page speed by minimizing HTTP requests, compressing images, and using a content delivery network (CDN).
- Monitor Your SEO Performance: Monitor your SEO performance using tools like Google Analytics and Google Search Console.
11. Security Best Practices
Security is paramount when developing web applications. Django provides several built-in security features that help protect your application from common web vulnerabilities. Here are some security best practices:
- Use HTTPS: Use HTTPS to encrypt all traffic between the user and your server.
- Protect Against CSRF: Enable CSRF protection to prevent cross-site request forgery attacks.
- Protect Against XSS: Sanitize user input to prevent cross-site scripting attacks.
- Use Secure Password Hashing: Use Django’s built-in password hashing to store passwords securely.
- Protect Against SQL Injection: Use Django’s ORM to prevent SQL injection attacks.
- Limit File Uploads: Limit file uploads to prevent malicious files from being uploaded to your server.
- Keep Django and Dependencies Up-to-Date: Keep Django and its dependencies up-to-date to patch security vulnerabilities.
- Monitor Your Application for Security Threats: Monitor your application for security threats using tools like Sentry and New Relic.
- Use a Content Security Policy (CSP): Use a CSP to control the resources that your browser is allowed to load.
- Implement Rate Limiting: Implement rate limiting to prevent brute-force attacks.
12. Deploying Your Django Application
Deploying your Django application involves making it accessible to users on the internet. Here are some common deployment options:
- Platform as a Service (PaaS): PaaS providers like Heroku, AWS Elastic Beanstalk, and Google App Engine simplify deployment by providing a managed environment.
- Virtual Private Server (VPS): VPS providers like DigitalOcean, Linode, and Vultr provide a virtual server that you can configure to host your Django application.
- Dedicated Server: A dedicated server provides you with full control over the hardware and software.
- Docker: Docker allows you to package your Django application and its dependencies into a container that can be easily deployed to any environment.
13. Tips and Tricks for Django Development
Here are some tips and tricks to enhance your Django development experience:
- Use Django Debug Toolbar: The Django Debug Toolbar provides valuable insights into your application’s performance.
- Use Django Extensions: Django Extensions provides useful management commands and tools.
- Use Django REST Framework: Django REST Framework simplifies the creation of RESTful APIs.
- Use Celery for Asynchronous Tasks: Celery allows you to run tasks asynchronously, improving your application’s performance.
- Use Caching: Caching can significantly improve your application’s performance by storing frequently accessed data in memory.
- Write Unit Tests: Writing unit tests ensures the reliability of your code.
- Use a Consistent Coding Style: Use a consistent coding style to improve readability and maintainability.
- Read the Django Documentation: The Django documentation is a valuable resource for learning about Django’s features and best practices.
- Contribute to the Django Community: Contribute to the Django community by submitting bug reports, contributing code, and answering questions on the Django forum.
- Use a Virtual Environment: Always use a virtual environment to isolate your project’s dependencies.
14. Real-World Examples of Django Projects
Django is used by many organizations to build a wide variety of web applications. Here are some real-world examples:
- Instagram: Instagram uses Django to power its backend.
- Mozilla: Mozilla uses Django to power its support website.
- Pinterest: Pinterest uses Django to power its recommendation engine.
- Bitbucket: Bitbucket uses Django to power its code repository.
- The Washington Post: The Washington Post uses Django to power its website.
15. Common Mistakes to Avoid
- Not Using a Virtual Environment: Always use a virtual environment to isolate your project’s dependencies.
- Not Using Version Control: Use version control (e.g., Git) to track changes to your code.
- Not Writing Unit Tests: Writing unit tests ensures the reliability of your code.
- Not Following Security Best Practices: Follow security best practices to protect your application from web vulnerabilities.
- Not Optimizing Performance: Optimize your application’s performance by using caching, asynchronous tasks, and other techniques.
- Not Reading the Documentation: The Django documentation is a valuable resource for learning about Django’s features and best practices.
- Overcomplicating Your Code: Keep your code simple and easy to understand.
- Ignoring Errors: Pay attention to errors and warnings, and fix them promptly.
- Not Keeping Django and Dependencies Up-to-Date: Keep Django and its dependencies up-to-date to patch security vulnerabilities and take advantage of new features.
16. Frequently Asked Questions (FAQ)
Here are some frequently asked questions about Django:
1. What is Django?
Django is a high-level Python web framework that encourages rapid development and clean, pragmatic design.
2. Why use Django?
Django provides a lot of built-in features, such as an ORM, template engine, and admin interface, which can save you a lot of time and effort.
3. How do I install Django?
You can install Django using pip: pip install django
.
4. What is a virtual environment?
A virtual environment is a tool that allows you to create isolated Python environments for each project.
5. What is a Django model?
A Django model is a Python class that represents a database table.
6. What is a Django view?
A Django view is a Python function that takes a web request and returns a web response.
7. What is a Django template?
A Django template is an HTML file with embedded code that allows dynamic content generation.
8. What is Django middleware?
Middleware is a framework of hooks into Django’s request/response processing.
9. How do I deploy a Django application?
You can deploy a Django application to a variety of platforms, such as Heroku, AWS, and Google Cloud Platform.
10. How can I contribute to Django?
You can contribute to Django by submitting bug reports, contributing code, and answering questions on the Django forum.
17. Resources for Further Learning
- Django Documentation: The official Django documentation is an invaluable resource.
- CONDUCT.EDU.VN Articles: Explore our articles for practical guidance and real-world examples.
- Django Tutorials: Many online tutorials offer step-by-step instructions for building Django applications.
- Django Books: Books like “Django for Beginners” and “Two Scoops of Django” provide in-depth knowledge.
- Django Community: Engage with the Django community through forums, mailing lists, and meetups.
Conclusion
This complete beginner’s guide to django part 2 has provided a solid foundation for building web applications with Django. Remember, continuous learning and practice are key to mastering Django. For more detailed guides, resources, and support, visit CONDUCT.EDU.VN at 100 Ethics Plaza, Guideline City, CA 90210, United States, or contact us via Whatsapp at +1 (707) 555-1234.
Ready to build your own amazing Django applications? Head over to conduct.edu.vn now for more in-depth tutorials, examples, and community support!